Study notes
Build a python app in isolation.
Solution: virtual environment
Self-contained copy of everything needed to run your program. This includes the Python interpreter and any libraries your program needs. By using a virtual environment, you can ensure your program will have access to the correct versions and resources to run correctly.
Steps:
- Create a virtual environment that won't affect the rest of the machine.
- Step into the virtual environment, where you specify the Python version and libraries that you need.
- Develop your program.
Create & go to app folder.
Run:
python -m venv env
Result a folders structure (may vary)
/env
|__/Include
|__/Lib
|_____site-packages
|__ /Scripts
Don't put your program files in the env directory. We suggest that you put your files in the srcdirectory or similar.
1.1. Activate the virtual environment
activate
#or
PATH_TO_ACTIVATE/activat
Result
(env) in front of prompt
To desactivateL
deactivate
ResultNo more (env) in front of prompt
1.2. Install a package
pip install python-dateutil
Once installed you will see
/
|__/env
|__/Lib
|__/dateutil
To see what packages are now installed in your virtual environmen
Run
pip freeze
Result:python-dateutil==2.8.2
...
More ways to install a package:
- Have a set of files on your machine and install from that source
cd <to where the package is on your machine>
python3 -m pip install . - Install from a GitHub repository
git+https://github.com/your-repo.git - Install from a compressed archive file
python3 -m pip install package.tar.gz
from datetime import *
from dateutil.relativedelta import *
now = datetime.now()
print(now)
now = now + relativedelta(months=1, weeks=1, hour=10)
print(now)
Distribute project to others for collaboration.
Create a project file
pip freeze > requirements.txt
Result:Creates a requirements.txtfile with all the packages that the program needs.
Create a .gitignorefile, and check in your application code and requirements.txt.
src/
requirements.txt
Check in the code to GitHub.Commit
Publish to GitHub
Check Online
Result - all files from requirements.txt are online (GitHub)
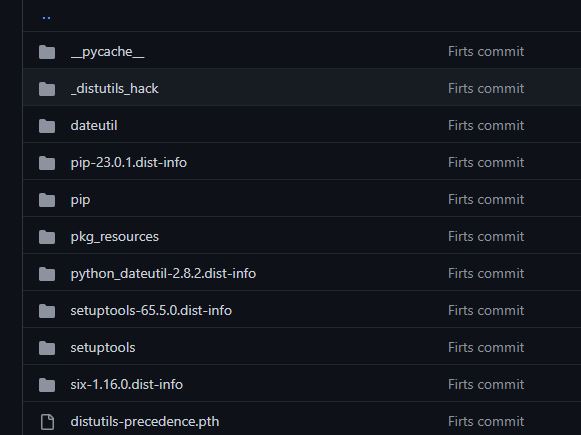
Consume a project
Create a folder
Go into
Get the git URL from GitHub, and run:
git clonehttps://github.com/corifeanu/Azure.git
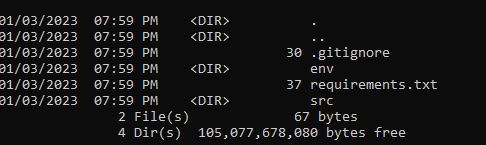
Install requirements.
Run:
pip install -r requirements.txt
Update/Run your all (what is in src).
Manage dependencies
Kee upgrading your packages:
- Bug fixes.
The library that you use might have problems. For example, a feature doesn't work as intended and the author goes in to fix it. You most likely want to upgrade the package as soon as such a fix is in place. - Security issues.
Your package might have a security vulnerability. After such a fix is released, you want to upgrade the package to protect your company and your customers. - Additional features.
The release of more features is nice, though it isn't a strong reason to upgrade the package. Still, if there's a feature that you've been waiting for, you might want to upgrade for that reason
Specific version
pip install python-dateutil===1.4
Check if version is availablepip install python-dateutil===randomwords
Result:ERROR: Could not find a version that satisfies the requirement python-dateutil===randomwords (from versions: 1.4, 1.4.1, 1.5, 2.1, 2.2, 2.3, 2.4.0, 2.4.1, 2.4.1.post1, 2.4.2, 2.5.0, 2.5.1, 2.5.2, 2.5.3, 2.6.0, 2.6.1, 2.7.0, 2.7.1, 2.7.2, 2.7.3, 2.7.4, 2.7.5, 2.8.0, 2.8.1, 2.8.2)
ERROR: No matching distribution found for python-dateutil===randomwords
Use:
pip freeze
Result...
python-dateutil==1.4
...
Install last one
pip installpython-dateutil===2.8.2
Upgrade
pip install python-dateutil --upgrade
Versioning plain English:
- The leftmost number is called Major. If this number increases, many things have changed, and you can no longer assume that methods are named the same or have the same number of arguments as before.
- The middle number is called Minor. If it changes, a feature has been added.
- The rightmost number is called Patch. If this number increases, it most likely means that a bug has been corrected.
pip install "python-dateutil==2.8.*" --upgrade
Remove one package only:
pip uninstall python-dateutil
Remove all installed packages, by first writing them to a requirements.txt list and then removing all packages in that list.
pip freeze > requirements.txt
pip uninstall -r requirements.txt -y
Now if you run pip freeze, you see that it contains only the following output:
pip-autoremove==0.10.0